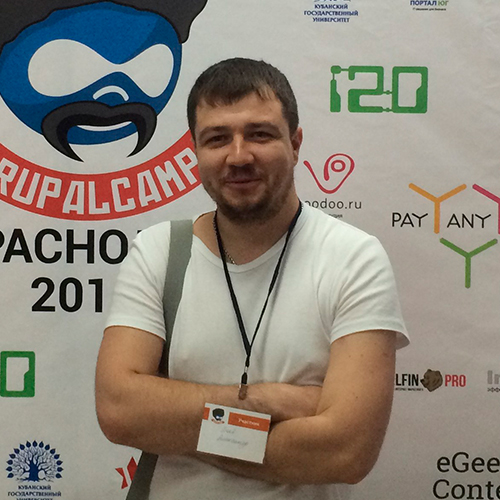
About me
Since 2009, I have been actively involved in developing websites on the Drupal CMS, professionally growing and honing my skills.
I specialize in Front-end development and have successfully implemented numerous projects, allowing me to delve deep into the intricacies and capabilities of this platform.
Additionally, I develop projects using React.js and Next.js, possessing substantial experience with these technologies. My professionalism and creative approach to each task enable me to create innovative and high-performance solutions, making me a valuable asset to any team.
Education
Sept. 2003 — Jun. 2004
Russia, Krasnodar
Professional Lyceum #41
Computer Operator
I completed courses on working with computers, and received the profession of a computer operator.
Oct. 2021
Russia, Moscow
University «Synergy»
Web development
Bachelor's degree, Non-state educational private institution of higher education «Moscow Financial and Industrial University «Synergy».
Certificates
I have been awarded certificates of distinction from the university for achieving high scores on exams, demonstrating my dedication and proficiency in key subject areas that are directly relevant to the position.
I have completed specialized courses in the ELMA365 system, which is an advanced cloud adaptation of the ELMA BPM system focused on the automation and robotization of business processes. Utilizing its Low-code platform, I have successfully obtained certificates demonstrating my ability to rapidly transform business needs into effective solutions, enhancing operational efficiencies.
I have successfully completed and received certifications on the Visiology platform, gaining advanced skills in data analysis and JavaScript development critical for effective data-driven decision-making.
As a certified expert in CMS 1C-Bitrix and Bitrix24, I have mastered all the necessary tools for effective digital management and development. My proven achievements, demonstrated by successfully passing all required certification tests, ensure that I am ready to immediately contribute to the success of your team. You can always verify my qualifications on the official 1C-Bitrix website using the provided certificate numbers.
Work experience
Web Developer at LLC ForwardMobile
I have extensive experience in remote programming of payment terminal interfaces and developing innovative functionalities for dealer accounts. My expertise spans across using programming languages such as Perl, HTML, Less, and CSS to enhance user experiences and streamline operations.
Web Developer at LLC LabSales
My experience encompasses website development on CMS Drupal versions 6 through 8, where I honed my skills in sophisticated web solutions. This role expanded my expertise to include developing on platforms such as 1C-Bitrix and CMS NetCat, enabling me to deliver versatile and robust websites tailored to specific business needs.
Website development at LLC Zwolves
At Zwolves, I honed my web development skills, focusing on creating corporate websites and online stores, and integrating websites with third-party resources. My work involved utilizing a comprehensive tech stack, including Drupal, Bootstrap, Twig, Less, Scss, Node.js, JavaScript, HTML & CSS, MySql, PHP, Symfony, Storybook, and tools like Git, Docker, and Atlassian suite (Bitbucket, Confluence, Jira, Trello), ensuring robust, scalable, and efficient digital solutions.
Web Developer at LTD ASB Studio
At ASB Studio, I managed and executed projects involving the development of websites from initial prototypes and design to full development on CMS Drupal versions 8 and 9. Leveraging my extensive tech stack, which includes Bootstrap, Twig, Less, Scss, Node.js, JavaScript, HTML & CSS, MySql, PHP, Symfony, Storybook, and tools like Git, Docker, and the Atlassian suite (Bitbucket, Confluence, Jira, Trello), I delivered highly customized and scalable digital solutions tailored to meet specific client needs.
Website development at LTD i20
At 'i20', my role involved the development of new e-commerce and corporate websites using CMS Drupal 9, as well as providing support for existing projects on CMS Drupal 7-9. I was also responsible for the development of simple modules, themes, and more complex module configurations for CMS Drupal. Additionally, I successfully managed the migration of projects from Drupal 7 to Drupal 9, integrating Vue.js to enhance interactive elements and user experience.
Website development at LTD 7 Red Lines
In my recent role, I engaged in the development of new projects on CMS Drupal 9-10, focusing on frontend development and theme customization. Utilizing a robust tech stack that includes JavaScript, TypeScript, React, and Vue.js, along with extensive use of Bootstrap 5, I crafted dynamic and responsive designs. My expertise in backend technologies and proficiency in DevOps tools like Docker and Kubernetes enabled me to deliver robust and scalable web solutions. Additionally, I integrated modern web functionalities using preprocessors to align with contemporary web standards.
Web Developer at LTD ASB Studio
At ASB Studio, I managed and executed projects involving the development of websites from initial prototypes and design to full development on CMS Drupal versions 8 and 9. Leveraging my extensive tech stack, which includes Bootstrap, Twig, Less, Scss, Node.js, JavaScript, HTML & CSS, MySql, PHP, Symfony, Storybook, and tools like Git, Docker, and the Atlassian suite (Bitbucket, Confluence, Jira, Trello), I delivered highly customized and scalable digital solutions tailored to meet specific client needs.
Code example typescript
import { validateOrReject, IsEmail, MinLength } from "class-validator"; import { plainToClass } from "class-transformer"; class User { @IsEmail() email: string; @MinLength(10) password: string; constructor(email: string, password: string) { this.email = email; this.password = password; } } async function validateUser(user: User) { try { await validateOrReject(user); console.log('Пользователь валиден.'); } catch (errors) { console.log('Обнаружены ошибки валидации:', errors); } } async function getUserFromDb<T>(id: string, type: { new (...args: any[]): T }): Promise<T | null> { const userData = { email: "example@example.com", password: "verystrongpassword" }; const user = plainToClass(type, userData); await validateUser(user); return user; } (async () => { const user = await getUserFromDb<User>('123', User); if (user) { console.log('Пользователь получен:', user); } })();
Code example React App
import React from 'react'; import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; import Navbar from './Navbar'; import WeatherForm from './WeatherForm'; import WeatherDisplay from './WeatherDisplay'; import About from './About'; import NotFound from './NotFound'; import { WeatherProvider } from './WeatherContext'; function App() { return ( <Router> <WeatherProvider> <Navbar /> <Switch> <Route path="/" exact component={WeatherForm} /> <Route path="/weather" component={WeatherDisplay} /> <Route path="/about" component={About} /> <Route component={NotFound} /> </Switch> </WeatherProvider> </Router> ); } export default App;
import React, { useState, useContext } from 'react'; import { WeatherContext } from './WeatherContext'; function WeatherForm() { const [city, setCity] = useState(''); const { fetchWeather } = useContext(WeatherContext); const handleSubmit = (e) => { e.preventDefault(); fetchWeather(city); }; return (); } export default WeatherForm;
import React, { useContext } from 'react'; import { WeatherContext } from './WeatherContext'; function WeatherDisplay() { const { weatherData } = useContext(WeatherContext); return ({weatherData ? (); } export default WeatherDisplay;) : ({weatherData.city}
Temperature: {weatherData.temperature} °C
Condition: {weatherData.condition}
No weather data available. Please enter a city.
)}
Code example React Native App «Todo List»
import React, { useState } from 'react'; import { StyleSheet, View, TextInput, Button, FlatList, Text } from 'react-native'; import TodoItem from './components/TodoItem'; export default function App() { const [todos, setTodos] = useState([]); const [text, setText] = useState(''); const addTodo = () => { setTodos([...todos, { key: Math.random().toString(), name: text }]); setText(''); }; const deleteTodo = key => { setTodos(todos.filter(todo => todo.key !== key)); }; return (); } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#fff', paddingTop: 50, paddingHorizontal: 20, }, input: { marginBottom: 10, paddingHorizontal: 8, paddingVertical: 6, borderBottomWidth: 1, borderBottomColor: '#ddd', } }); ( )} />
import React from 'react'; import { StyleSheet, Text, TouchableOpacity, View } from 'react-native'; const TodoItem = ({ item, deleteTodo }) => { return (deleteTodo(item.key)}> ); }; const styles = StyleSheet.create({ item: { padding: 16, marginTop: 16, borderColor: '#bbb', borderWidth: 1, borderStyle: 'dashed', borderRadius: 10, } }); export default TodoItem;{item.name}
Example code Symfony
namespace App\Controller; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\Security\Http\Authentication\AuthenticationUtils; use App\Entity\User; use Doctrine\ORM\EntityManagerInterface; use Symfony\Component\Validator\Validator\ValidatorInterface; use App\Form\UserType; class DemoController extends AbstractController { /** * @Route("/", name="home") */ public function index(AuthenticationUtils $authenticationUtils): Response { $error = $authenticationUtils->getLastAuthenticationError(); $lastUsername = $authenticationUtils->getLastUsername(); return $this->render('index.html.twig', ['last_username' => $lastUsername, 'error' => $error]); } /** * @Route("/user/new", name="new_user") */ public function newUser(Request $request, EntityManagerInterface $em, ValidatorInterface $validator): Response { $user = new User(); $form = $this->createForm(UserType::class, $user); $form->handleRequest($request); if ($form->isSubmitted() && $form->isValid()) { $em->persist($user); $em->flush(); $errors = $validator->validate($user); if (count($errors) > 0) { return $this->render('user/new.html.twig', [ 'form' => $form->createView(), 'errors' => $errors, ]); } return $this->redirectToRoute('home'); } return $this->render('user/new.html.twig', [ 'form' => $form->createView(), ]); } /** * @Route("/api/users", methods="GET") */ public function getUsers(EntityManagerInterface $em): Response { $users = $em->getRepository(User::class)->findAll(); return $this->json($users); } }
Example of a MySql query
SELECT DISTINCT u.id, SUBSTRING_INDEX(u.phone, ':', 1) AS phone, u.first_name, u.second_name, u.updated_at FROM users u JOIN schools_users su ON u.id = su.user_id JOIN abonements a ON u.id = a.user_id LEFT JOIN children c ON u.id = c.user_id WHERE su.school_id = 3 AND a.abonement_type_id IS NOT NULL ORDER BY u.updated_at DESC
Projects
My task was to develop a frontend based on the layout, and build a website on CMS Drupal.
in some projects to develop a module for CMS Drupal.